Simulation Controller module
Class diagram
![digraph "classes_sim_con" {
rankdir=BT
charset="utf-8"
"DMT.core.data_frame.DataFrame" [color="black", fontcolor="black", label="DataFrame", shape="record", style="solid"];
"DMT.core.DutView" [color="black", fontcolor="black", label="DutView", shape="record", style="solid"];
"scp.SCPClient" [color="black", fontcolor="black", label="SCPClient", shape="record", style="solid"];
"paramiko.client.SSHClient" [color="black", fontcolor="black", label="SSHClient", shape="record", style="solid"];
"DMT.core.SimCon" [color="black", fontcolor="black", label="SimCon", shape="record", style="solid"];
"DMT.core.naming.SpecifierStr" [color="black", fontcolor="black", label="SpecifierStr", shape="record", style="solid"];
"DMT.core.Sweep" [color="black", fontcolor="black", label="Sweep", shape="record", style="solid"];
"DMT.core.sweep.SweepDef" [color="black", fontcolor="black", label="SweepDef", shape="record", style="solid"];
"DMT.core.data_frame.DataFrame" -> "DMT.core.Sweep" [arrowhead="diamond", arrowtail="none", fontcolor="darkgreen", label="df", style="solid"];
"DMT.core.naming.SpecifierStr" -> "DMT.core.sweep.SweepDef" [arrowhead="diamond", arrowtail="none", fontcolor="darkgreen", label="var_name", style="solid"];
"paramiko.client.SSHClient" -> "DMT.core.SimCon" [arrowhead="diamond", arrowtail="none", fontcolor="darkgreen", label="ssh_client", style="solid"];
"scp.SCPClient" -> "DMT.core.SimCon" [arrowhead="diamond", arrowtail="none", fontcolor="darkgreen", label="scp_client", style="solid"];
"DMT.core.sweep.SweepDef" -> "DMT.core.Sweep" [arrowhead="diamond", arrowtail="none", fontcolor="darkgreen", label="sweepdefs", style="solid", taillabel="0..*"];
"DMT.core.Sweep" -> "DMT.core.SimCon" [arrowhead="diamond", arrowtail="none", fontcolor="darkgreen", label="sim_list['sweep']", style="solid", taillabel="0..*"];
"DMT.core.DutView" -> "DMT.core.SimCon" [arrowhead="diamond", arrowtail="none", fontcolor="darkgreen", label="sim_list['dut']", style="solid", taillabel="0..*"];
}](../_images/graphviz-4f20f9d590da4841f32bdbb4f7ddcba00f8773ee.png)
See the DutView documentation for available interfaces.
Sequenz for many simulations in parallel
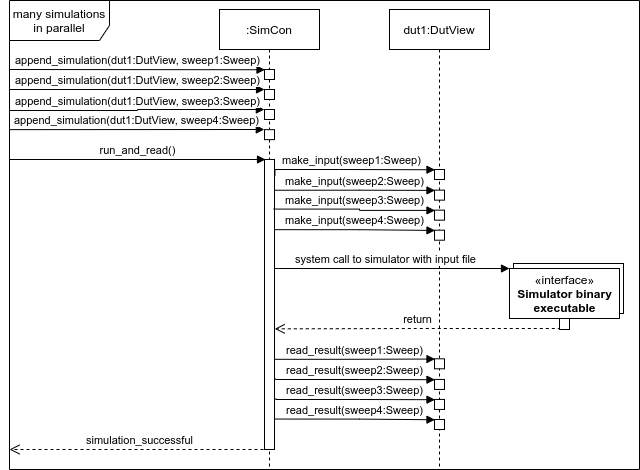
This Sequenz diagram shows how to use SimCon to run many simulations in parallel.
sim_con Module documentation
This module supplies a class to manage all simulations, regardless of the device simulator.
It works together with the DutView class, which is the subclass of all device simulators. Features:
Manages all simulations in a unified way, independent of the actual simulation backend.
Supports to run simulations on multiple cores in parallel
Supports to run simulations on a remote server (including file up- and download)
Author: Mario Krattenmacher | mario.krattenmacher@semimod.de Author: Markus Müller | markus.mueller@semimod.de
- class DMT.core.sim_con.SimCon(*args, **kwargs)[source]
Bases:
object
Simulation controller class. SINGLETON design pattern.
- Parameters:
- Attributes:
- n_core
int
Number of cores that shall be used for simulations.
- t_max
float
Timeout for simulations. If a simulation runs longer than t_max in seconds, it is killed.
- sim_list[{‘dut’:
DutView
, ‘sweep’:Sweep
}] A list of dicts containing the queued simulations. Each dict holds a ‘dut’ key value pair and a ‘sweep’ key value pair.
- ssh_client
Client to execute SSH commands on a remote server.
- scp_client
Client to transfer files to a remote server via SCP.
- n_core
- append_simulation(dut: List[DutView] | DutView | None = None, sweep: List[Sweep] | Sweep | None = None)[source]
Adds DutViews together with Sweeps to the list of simulations sim_list.
This methods adds each dut with a copy of each sweep to the simulation list.
- copy_from_server(dut, sweep, zip_result=True)[source]
Collects the simulation data from the server.
- Parameters:
- dut
DutView
- sweep
Sweep
- zip_resultbool,
optional
If True, the result is zipped before transfer, the zip is copied and then unzipped locally.
- dut
- copy_log_from_server(dut, sweep)[source]
Collects the simulation log file from the server.
- Parameters:
- dut
DutView
- sweep
Sweep
- dut
- copy_zip_to_server(sims_to_zip)[source]
Copies the simulation data to the server. Before doing this, old simulation data is deleted
- run_and_read(force=False, remove_simulations=False, parallel_read=False, validate=True)[source]
Run all queued simulations and load the results into the Duts’ databases.
- Parameters:
- forcebool,
optional
If True, the simulations will be run and saved back. If False, the simulations will only be run if that has not already been done before. This is ensured using the hash system., by default False
- remove_simulationsbool,
optional
If True, the simulation results will be deleted after read in, by default False. Activate to save disk space.
- parallel_readbool,
optional
If True, the simulation results are read in using joblib parallel, by default False. Is False because some simulators have issues with this…
- forcebool,
- Returns: