Running a Simulation and Plotting
In DMT
simulators are interfaced using the abstract classes DutCircuit and DutTcad. These classes define the missing methods which are needed to run any simulator either installed on your pc or on a remote server available using ftp and ssh. The interface uses always the same steps which are shown in the following image:
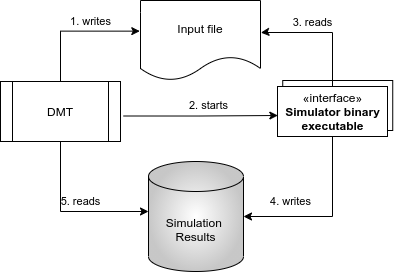
A simulation controller SimCon instance calls the
make_input
method of the dut to simulate. This will then write the input file in a simulation folder. The folder is created in the simulation directory of the Configuration:directories[simulations]/dut_folder/sim_folder
. The dut folder name consist of the dut name appended by the unique dut hash. The sim folder consist of the sweep name and the unique sweep hash. This way, no simulation has to be run twice.The simulator binary is called using a sys call with the input file as a parameter in the simulation folder.
The simulator reads the input file and executes the simulation accordingly. If it returns a non-zero exit value, the
simulation_successful
return value is set toFalse
.The simulator writes the simulation results into the simulation folder. This is often done in proprietary binary formats or at least with sorting and naming unknown to DMT.
For this the
read_result
method of the simulated dut is called to read and convert the results into the DMT format. If the simulation binary returned a non-zero exit value, the result is read anyway. Yes we know… but we tested many simulators, and they just behave strange…
After these steps the simulation results are ready to be used. An example code how to make a circuit simulation is shown in the following.
The code for the simulation
import types
import numpy as np
from pathlib import Path
from DMT.core import Sweep, SimCon, Plot, specifiers, DutType, MCard
from DMT.core.circuit import Circuit, CircuitElement, HICUML2_HBT, SHORT, VOLTAGE, RESISTANCE
from DMT.xyce import DutXyce
from DMT.ngspice import DutNgspice
# path to DMT test cases
path_test = Path(__file__).resolve().parent.parent.parent.parent / "test"
# load a hicum/L2 modelcard library by loading the corresponding *va code.
modelcard = MCard(
["C", "B", "E", "S", "T"],
default_module_name="",
default_subckt_name="",
va_file=path_test / "test_interface_xyce" / "hicuml2v2p4p0_xyce.va",
)
modelcard.load_model_parameters(
path_test / "test_core_no_interfaces" / "test_modelcards" / "npn_full.lib",
)
modelcard.update_from_vae(remove_old_parameters=True)
# bind the correct get_circuit method in order to make it easily available for simulation
def get_circuit(self):
"""
Parameter
------------
modelcard : :class:`~DMT.core.MCard`
Returns
-------
circuit : :class:`~DMT.core.circuit.Circuit`
"""
circuit_elements = []
# model instance
circuit_elements.append(
CircuitElement(
"hicumL2_test",
"Q_H",
[f"n_{node.upper()}" for node in self.nodes_list],
parameters=self,
)
)
# BASE NODE CONNECTION #############
# shorts for current measurement
circuit_elements.append(CircuitElement(SHORT, "I_B", ["n_B", "n_B_FORCED"]))
# COLLECTOR NODE CONNECTION #############
circuit_elements.append(CircuitElement(SHORT, "I_C", ["n_C", "n_C_FORCED"]))
# EMITTER NODE CONNECTION #############
circuit_elements.append(CircuitElement(SHORT, "I_E", ["n_E", "0"]))
# add sources and thermal resistance
circuit_elements.append(
CircuitElement(
VOLTAGE, "V_B", ["n_B_FORCED", "0"], parameters=[("Vdc", "V_B"), ("Vac", "V_B_ac")]
)
)
circuit_elements.append(
CircuitElement(
VOLTAGE, "V_C", ["n_C_FORCED", "0"], parameters=[("Vdc", "V_C"), ("Vac", "V_C_ac")]
)
)
circuit_elements += ["V_B=0", "V_C=0", "ac_switch=0", "V_B_ac=1-ac_switch", "V_C_ac=ac_switch"]
return Circuit(circuit_elements)
modelcard.get_circuit = types.MethodType(get_circuit, modelcard)
duts = []
# init an Xyce Dut
duts.append(DutXyce(None, DutType.npn, modelcard, nodes="C,B,E,S,T", reference_node="E"))
# DMT uses the exact same interface for all circuit simulators, e.g. the call for a ngspice simulation would be:
duts.append(DutNgspice(None, DutType.npn, modelcard, nodes="C,B,E,S,T", reference_node="E"))
# isn't it great?!?
# create a sweep (all DMT Duts can use this!)
# some column names we want to simulate and plot
col_ve = specifiers.VOLTAGE + "E"
col_vb = specifiers.VOLTAGE + "B"
col_vc = specifiers.VOLTAGE + "C"
col_vbe = specifiers.VOLTAGE + ["B", "E"]
col_vcb = specifiers.VOLTAGE + ["C", "B"]
col_vbc = specifiers.VOLTAGE + ["B", "C"]
col_ic = specifiers.CURRENT + "C"
col_freq = specifiers.FREQUENCY
col_ft = specifiers.TRANSIT_FREQUENCY
sweepdef = [
{"var_name": col_freq, "sweep_order": 4, "sweep_type": "LIST", "value_def": [10e9]},
{"var_name": col_vb, "sweep_order": 3, "sweep_type": "LIN", "value_def": [0.5, 1, 51]},
{
"var_name": col_vc,
"sweep_order": 3,
"sweep_type": "SYNC",
"master": col_vb,
"offset": col_vcb,
},
{"var_name": col_vcb, "sweep_order": 2, "sweep_type": "LIST", "value_def": [-0.5, 0, 0.5]},
{"var_name": col_ve, "sweep_order": 1, "sweep_type": "CON", "value_def": [0]},
]
outputdef = ["I_C", "I_B"]
othervar = {"TEMP": 300}
sweep = Sweep("gummel", sweepdef=sweepdef, outputdef=outputdef, othervar=othervar)
# The simulation controller can control all simulators implemented in DMT!
sim_con = SimCon()
# Add the desired simulation to the queue and start the simulation
sim_con.append_simulation(dut=duts, sweep=sweep)
sim_con.run_and_read(force=True, remove_simulations=False)
# Plot and save as pdf
plt_ic = Plot(
plot_name="J_C(V_BE)",
x_specifier=col_vbe,
y_specifier=col_ic,
y_scale=1e3,
y_log=True,
legend_location="lower right",
)
plt_ft = Plot(
plot_name="F_T(J_C)",
x_specifier=col_ic,
x_scale=1e3,
x_log=True,
y_specifier=col_ft,
legend_location="upper left",
)
for dut in duts:
name = dut.name.split("_")[0] + " "
# Read back the iv data of the circuit simulator
data = dut.get_data(sweep=sweep)
# Ensure derived quantities, e.g. the circuit simulator only gives you S parameters
data.ensure_specifier_column(col_vbe, ports=["B", "C"])
data.ensure_specifier_column(col_vbc, ports=["B", "C"])
data.ensure_specifier_column(col_ft, ports=["B", "C"])
for i_vbc, vbc, data_vbc in data.iter_unique_col(col_vbc, decimals=3):
vbc = np.real(vbc)
plt_ic.add_data_set(
data_vbc[col_vbe], data_vbc[col_ic], label=name + col_vbc.to_legend_with_value(vbc)
)
plt_ft.add_data_set(
data_vbc[col_ic], data_vbc[col_ft], label=name + col_vbc.to_legend_with_value(vbc)
)
plt_ic.x_limits = 0.7, 1
plt_ic.y_limits = 1e-2, 1e2
plt_ft.x_limits = 1e-2, 1e2
plt_ft.y_limits = 0, 420
plt_ic.plot_pyqtgraph(show=False)
plt_ft.plot_pyqtgraph()
# plt_ic.save_tikz(
# Path(__file__).parent.parent / "_static" / "running_a_simulation",
# standalone=True,
# build=True,
# clean=True,
# width="3in",
# )
# plt_ft.save_tikz(
# Path(__file__).parent.parent / "_static" / "running_a_simulation",
# standalone=True,
# build=True,
# clean=True,
# width="3in",
# )
The generated files
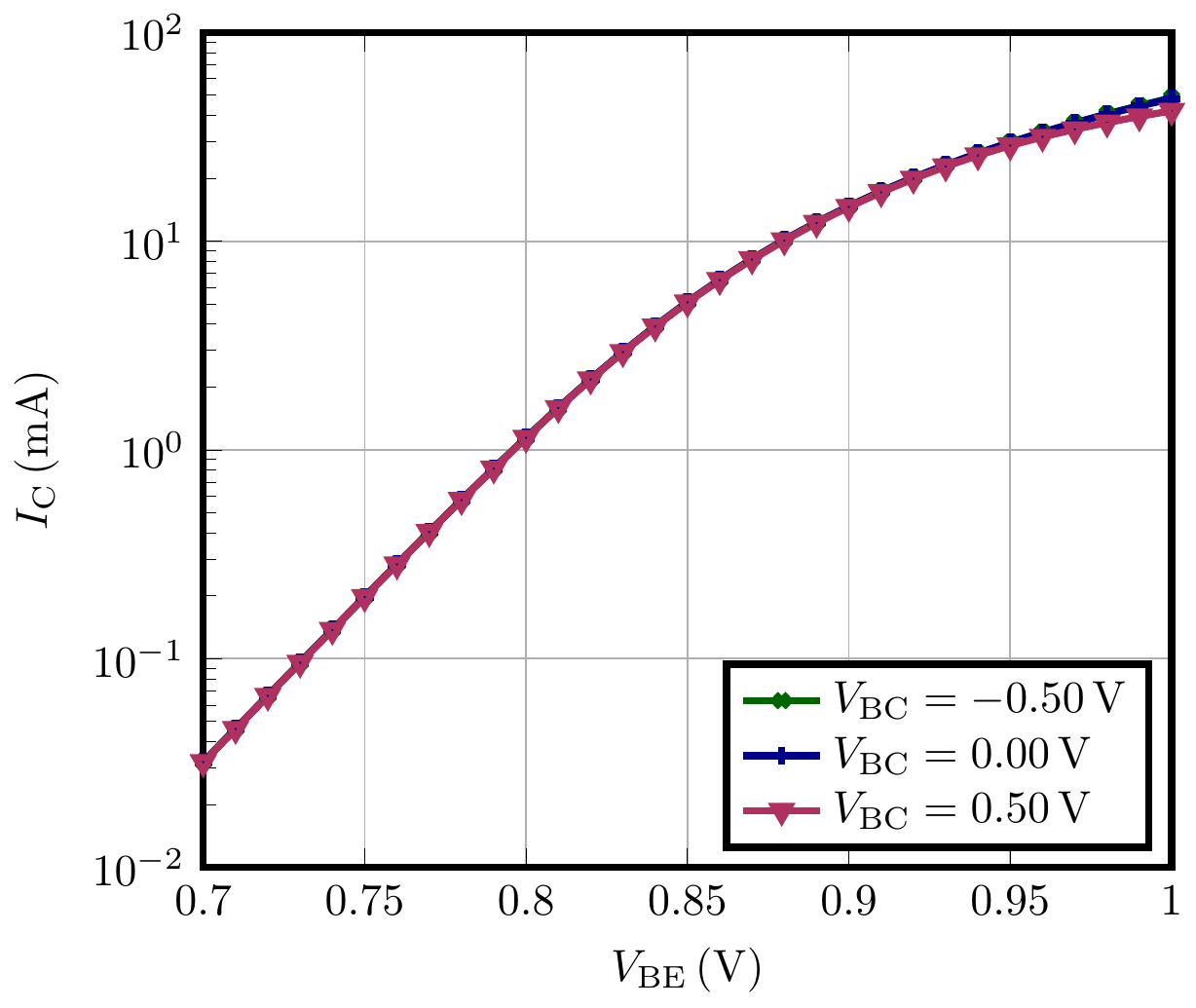
1\documentclass[class=IEEEtran]{standalone}
2\usepackage{tikz,amsmath,siunitx}
3\usetikzlibrary{arrows,snakes,backgrounds,patterns,matrix,shapes,fit,calc,shadows,plotmarks}
4\usepackage[graphics,tightpage,active]{preview}
5\usepackage{pgfplots}
6\pgfplotsset{compat=newest}
7\usetikzlibrary{shapes.geometric}
8\PreviewEnvironment{tikzpicture}
9\PreviewEnvironment{equation}
10\PreviewEnvironment{equation*}
11\newlength\figurewidth
12\newlength\figureheight
13\begin{document}
14\setlength\figurewidth{60mm}
15\setlength\figureheight{60mm}
16\begin{tikzpicture}[font=\normalsize]
17\pgfplotsset{every axis/.append style={ultra thick},compat=1.5},
18\definecolor{color0}{rgb}{0.00000, 0.39216, 0.00000}
19\definecolor{color1}{rgb}{0.00000, 0.00000, 0.54510}
20\definecolor{color2}{rgb}{0.69020, 0.18824, 0.37647}
21
22\begin{axis}[scale only axis,ytick pos=left,
23width=3in,
24xlabel={$V_{\mathrm{BE}}\left(\si{\volt}\right)$},
25ylabel={$I_{\mathrm{C}}\left(\si{\milli\ampere}\right)$},
26ymode=log,
27xmin=0.7,
28xmax=1,
29restrict x to domain=0.14:5,
30log basis x=10,
31ymin=0.01,
32ymax=100,
33restrict y to domain=-10:10,
34log basis y=10,
35xmajorgrids,
36enlargelimits=false,
37scaled ticks=false,
38ymajorgrids,
39x tick style={color=black},
40y tick style={color=black},
41x grid style={white!69.01960784313725!black},
42y grid style={white!69.01960784313725!black},
43/tikz/mark repeat=1,
44legend style={at={(0.98,0.02)}, anchor=south east,legend cell align=left, align=left},
45]
46\addplot [color=color0, solid, mark=x, mark options={solid}, mark phase=1, ]
47 table[row sep=crcr, x expr=\thisrowno{0}*1.000000e+00, y expr=\thisrowno{1}*1.000000e+03]{
480.5 1.80639e-08\\
490.51 2.63886e-08\\
500.52 3.85387e-08\\
510.53 5.62658e-08\\
520.54 8.21192e-08\\
530.55 1.19808e-07\\
540.56 1.74723e-07\\
550.57 2.54696e-07\\
560.58 3.71093e-07\\
570.59 5.40396e-07\\
580.6 7.8648e-07\\
590.61 1.1439e-06\\
600.62 1.66263e-06\\
610.63 2.41486e-06\\
620.64 3.50476e-06\\
630.65 5.08248e-06\\
640.66 7.36121e-06\\
650.67 1.06546e-05\\
660.68 1.54062e-05\\
670.69 2.22549e-05\\
680.7 3.21173e-05\\
690.71 4.63085e-05\\
700.72 6.67132e-05\\
710.73 9.60261e-05\\
720.74 0.00013808\\
730.75 0.000198284\\
740.76 0.000284178\\
750.77 0.000406103\\
760.78 0.000577911\\
770.79 0.000817588\\
780.8 0.00114752\\
790.81 0.00159412\\
800.82 0.00218643\\
810.83 0.00295385\\
820.84 0.00392323\\
830.85 0.00511616\\
840.86 0.00654728\\
850.87 0.00822382\\
860.88 0.0101462\\
870.89 0.0123096\\
880.9 0.0147051\\
890.91 0.0173211\\
900.92 0.0201448\\
910.93 0.0231623\\
920.94 0.0263597\\
930.95 0.0297231\\
940.96 0.0332389\\
950.97 0.0368942\\
960.98 0.0406763\\
970.99 0.0445729\\
981 0.0485721\\
99};
100\addlegendentry{$V_{\mathrm{BC}}=\SI{-0.50}{\volt}$}
101\addplot [color=color1, solid, mark=+, mark options={solid}, mark phase=1, ]
102 table[row sep=crcr, x expr=\thisrowno{0}*1.000000e+00, y expr=\thisrowno{1}*1.000000e+03]{
1030.5 1.80136e-08\\
1040.51 2.6316e-08\\
1050.52 3.8434e-08\\
1060.53 5.61146e-08\\
1070.54 8.19008e-08\\
1080.55 1.19492e-07\\
1090.56 1.74267e-07\\
1100.57 2.54038e-07\\
1110.58 3.70145e-07\\
1120.59 5.3903e-07\\
1130.6 7.84516e-07\\
1140.61 1.14108e-06\\
1150.62 1.65858e-06\\
1160.63 2.40904e-06\\
1170.64 3.49644e-06\\
1180.65 5.07059e-06\\
1190.66 7.34427e-06\\
1200.67 1.06304e-05\\
1210.68 1.5372e-05\\
1220.69 2.22064e-05\\
1230.7 3.20488e-05\\
1240.71 4.62117e-05\\
1250.72 6.65771e-05\\
1260.73 9.58352e-05\\
1270.74 0.000137814\\
1280.75 0.000197914\\
1290.76 0.000283668\\
1300.77 0.000405407\\
1310.78 0.000576977\\
1320.79 0.000816361\\
1330.8 0.00114596\\
1340.81 0.00159219\\
1350.82 0.00218417\\
1360.83 0.00295136\\
1370.84 0.00392066\\
1380.85 0.00511375\\
1390.86 0.00654528\\
1400.87 0.00822245\\
1410.88 0.0101457\\
1420.89 0.0123098\\
1430.9 0.0147058\\
1440.91 0.0173218\\
1450.92 0.0201443\\
1460.93 0.0231587\\
1470.94 0.0263498\\
1480.95 0.0297021\\
1490.96 0.0331992\\
1500.97 0.0368232\\
1510.98 0.0405517\\
1520.99 0.0443502\\
1531 0.0481583\\
154};
155\addlegendentry{$V_{\mathrm{BC}}=\SI{0.00}{\volt}$}
156\addplot [color=color2, solid, mark=triangle, mark options={solid, rotate=180}, mark phase=1, ]
157 table[row sep=crcr, x expr=\thisrowno{0}*1.000000e+00, y expr=\thisrowno{1}*1.000000e+03]{
1580.5 9.27458e-10\\
1590.51 7.4876e-09\\
1600.52 1.97108e-08\\
1610.53 3.74843e-08\\
1620.54 6.33443e-08\\
1630.55 1.0098e-07\\
1640.56 1.55755e-07\\
1650.57 2.35462e-07\\
1660.58 3.51408e-07\\
1670.59 5.19993e-07\\
1680.6 7.64977e-07\\
1690.61 1.12075e-06\\
1700.62 1.63703e-06\\
1710.63 2.38569e-06\\
1720.64 3.47042e-06\\
1730.65 5.0407e-06\\
1740.66 7.30873e-06\\
1750.67 1.05868e-05\\
1760.68 1.53167e-05\\
1770.69 2.21346e-05\\
1780.7 3.19532e-05\\
1790.71 4.60822e-05\\
1800.72 6.63988e-05\\
1810.73 9.55863e-05\\
1820.74 0.000137462\\
1830.75 0.00019741\\
1840.76 0.000282939\\
1850.77 0.000404337\\
1860.78 0.000575387\\
1870.79 0.000813969\\
1880.8 0.00114233\\
1890.81 0.00158668\\
1900.82 0.00217581\\
1910.83 0.00293882\\
1920.84 0.00390223\\
1930.85 0.00508728\\
1940.86 0.0065082\\
1950.87 0.00817167\\
1960.88 0.0100772\\
1970.89 0.0122182\\
1980.9 0.0145821\\
1990.91 0.0171503\\
2000.92 0.0198959\\
2010.93 0.0227786\\
2020.94 0.0257388\\
2030.95 0.0286968\\
2040.96 0.0315715\\
2050.97 0.0343251\\
2060.98 0.0369758\\
2070.99 0.0395621\\
2081 0.0421177\\
209};
210\addlegendentry{$V_{\mathrm{BC}}=\SI{0.50}{\volt}$}
211\end{axis}
212
213\end{tikzpicture}
214\end{document}
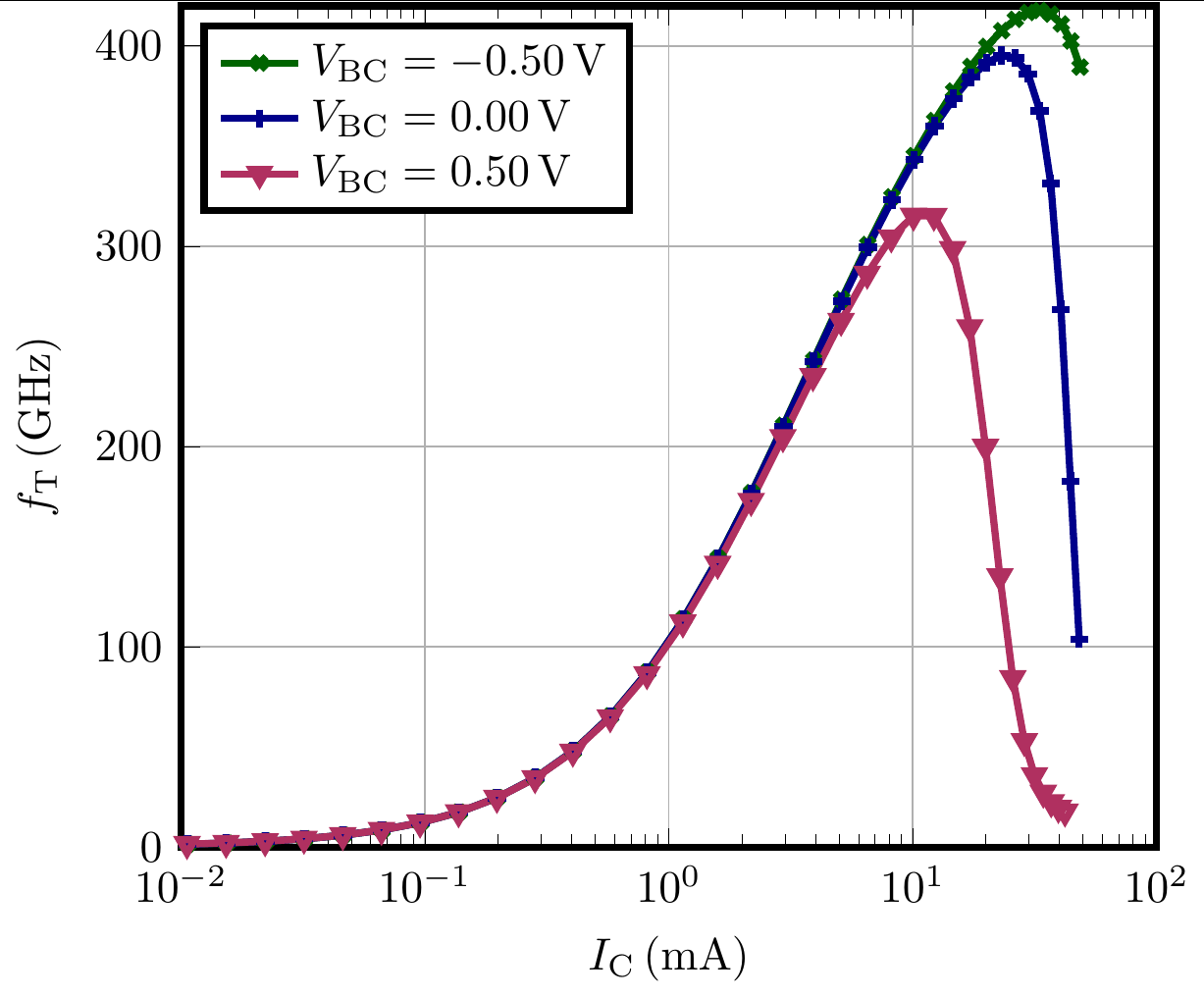
1\documentclass[class=IEEEtran]{standalone}
2\usepackage{tikz,amsmath,siunitx}
3\usetikzlibrary{arrows,snakes,backgrounds,patterns,matrix,shapes,fit,calc,shadows,plotmarks}
4\usepackage[graphics,tightpage,active]{preview}
5\usepackage{pgfplots}
6\pgfplotsset{compat=newest}
7\usetikzlibrary{shapes.geometric}
8\PreviewEnvironment{tikzpicture}
9\PreviewEnvironment{equation}
10\PreviewEnvironment{equation*}
11\newlength\figurewidth
12\newlength\figureheight
13\begin{document}
14\setlength\figurewidth{60mm}
15\setlength\figureheight{60mm}
16\begin{tikzpicture}[font=\normalsize]
17\pgfplotsset{every axis/.append style={ultra thick},compat=1.5},
18\definecolor{color0}{rgb}{0.00000, 0.39216, 0.00000}
19\definecolor{color1}{rgb}{0.00000, 0.00000, 0.54510}
20\definecolor{color2}{rgb}{0.69020, 0.18824, 0.37647}
21
22\begin{axis}[scale only axis,ytick pos=left,
23width=3in,
24xlabel={$I_{\mathrm{C}}\left(\si{\milli\ampere}\right)$},
25ylabel={$f_{\mathrm{T}}\left(\si{\giga\hertz}\right)$},
26xmode=log,
27xmin=0.01,
28xmax=100,
29restrict x to domain=-10:10,
30log basis x=10,
31ymin=0,
32ymax=420,
33restrict y to domain=0:2100,
34log basis y=10,
35xmajorgrids,
36enlargelimits=false,
37scaled ticks=false,
38ymajorgrids,
39x tick style={color=black},
40y tick style={color=black},
41x grid style={white!69.01960784313725!black},
42y grid style={white!69.01960784313725!black},
43/tikz/mark repeat=1,
44legend style={at={(0.02,0.98)}, anchor=north west,legend cell align=left, align=left},
45]
46\addplot [color=color0, solid, mark=x, mark options={solid}, mark phase=1, ]
47 table[row sep=crcr, x expr=\thisrowno{0}*1.000000e+03, y expr=\thisrowno{1}*1.000000e-09]{
481.80639e-08 9.26724e+10\\
492.63886e-08 6.116e+10\\
503.85387e-08 4.07307e+10\\
515.62658e-08 2.72951e+10\\
528.21192e-08 1.83731e+10\\
531.19808e-07 1.24098e+10\\
541.74723e-07 8.40734e+09\\
552.54696e-07 5.71522e+09\\
563.71093e-07 3.90476e+09\\
575.40396e-07 2.69183e+09\\
587.8648e-07 1.88791e+09\\
591.1439e-06 1.36868e+09\\
601.66263e-06 1.05367e+09\\
612.41486e-06 8.93344e+08\\
623.50476e-06 8.6136e+08\\
635.08248e-06 9.50402e+08\\
647.36121e-06 1.16982e+09\\
651.06546e-05 1.54797e+09\\
661.54062e-05 2.13321e+09\\
672.22549e-05 3.00092e+09\\
683.21173e-05 4.26251e+09\\
694.63085e-05 6.07866e+09\\
706.67132e-05 8.67614e+09\\
719.60261e-05 1.23675e+10\\
720.00013808 1.75706e+10\\
730.000198284 2.48224e+10\\
740.000284178 3.47723e+10\\
750.000406103 4.81356e+10\\
760.000577911 6.55815e+10\\
770.000817588 8.7547e+10\\
780.00114752 1.14004e+11\\
790.00159412 1.44281e+11\\
800.00218643 1.77057e+11\\
810.00295385 2.10612e+11\\
820.00392323 2.43229e+11\\
830.00511616 2.73564e+11\\
840.00654728 3.00814e+11\\
850.00822382 3.24687e+11\\
860.0101462 3.45246e+11\\
870.0123096 3.62742e+11\\
880.0147051 3.77484e+11\\
890.0173211 3.8975e+11\\
900.0201448 3.99749e+11\\
910.0231623 4.07587e+11\\
920.0263597 4.1327e+11\\
930.0297231 4.16694e+11\\
940.0332389 4.17661e+11\\
950.0368942 4.15882e+11\\
960.0406763 4.10984e+11\\
970.0445729 4.02459e+11\\
980.0485721 3.89436e+11\\
99};
100\addlegendentry{$V_{\mathrm{BC}}=\SI{-0.50}{\volt}$}
101\addplot [color=color1, solid, mark=+, mark options={solid}, mark phase=1, ]
102 table[row sep=crcr, x expr=\thisrowno{0}*1.000000e+03, y expr=\thisrowno{1}*1.000000e-09]{
1031.80136e-08 1.18811e+11\\
1042.6316e-08 7.83898e+10\\
1053.8434e-08 5.2163e+10\\
1065.61146e-08 3.49168e+10\\
1078.19008e-08 2.3472e+10\\
1081.19492e-07 1.58298e+10\\
1091.74267e-07 1.07058e+10\\
1102.54038e-07 7.26219e+09\\
1113.70145e-07 4.94724e+09\\
1125.3903e-07 3.39509e+09\\
1137.84516e-07 2.36284e+09\\
1141.14108e-06 1.68982e+09\\
1151.65858e-06 1.27113e+09\\
1162.40904e-06 1.04075e+09\\
1173.49644e-06 9.61219e+08\\
1185.07059e-06 1.01778e+09\\
1197.34427e-06 1.21485e+09\\
1201.06304e-05 1.57731e+09\\
1211.5372e-05 2.15124e+09\\
1222.22064e-05 3.01038e+09\\
1233.20488e-05 4.26494e+09\\
1244.62117e-05 6.0746e+09\\
1256.65771e-05 8.6653e+09\\
1269.58352e-05 1.23487e+10\\
1270.000137814 1.75416e+10\\
1280.000197914 2.47799e+10\\
1290.000283668 3.47112e+10\\
1300.000405407 4.80487e+10\\
1310.000576977 6.54589e+10\\
1320.000816361 8.73747e+10\\
1330.00114596 1.13764e+11\\
1340.00159219 1.4395e+11\\
1350.00218417 1.76607e+11\\
1360.00295136 2.10013e+11\\
1370.00392066 2.4245e+11\\
1380.00511375 2.72568e+11\\
1390.00654528 2.99559e+11\\
1400.00822245 3.23104e+11\\
1410.0101457 3.43216e+11\\
1420.0123098 3.60052e+11\\
1430.0147058 3.73758e+11\\
1440.0173218 3.84361e+11\\
1450.0201443 3.91677e+11\\
1460.0231587 3.95214e+11\\
1470.0263498 3.93989e+11\\
1480.0297021 3.8609e+11\\
1490.0331992 3.67653e+11\\
1500.0368232 3.31384e+11\\
1510.0405517 2.68355e+11\\
1520.0443502 1.82719e+11\\
1530.0481583 1.03973e+11\\
154};
155\addlegendentry{$V_{\mathrm{BC}}=\SI{0.00}{\volt}$}
156\addplot [color=color2, solid, mark=triangle, mark options={solid, rotate=180}, mark phase=1, ]
157 table[row sep=crcr, x expr=\thisrowno{0}*1.000000e+03, y expr=\thisrowno{1}*1.000000e-09]{
1589.27458e-10 1.64549e+11\\
1597.4876e-09 1.0934e+11\\
1601.97108e-08 7.30099e+10\\
1613.74843e-08 4.89271e+10\\
1626.33443e-08 3.28787e+10\\
1631.0098e-07 2.21443e+10\\
1641.55755e-07 1.49455e+10\\
1652.35462e-07 1.01101e+10\\
1663.51408e-07 6.8613e+09\\
1675.19993e-07 4.68234e+09\\
1687.64977e-07 3.22929e+09\\
1691.12075e-06 2.27372e+09\\
1701.63703e-06 1.66525e+09\\
1712.38569e-06 1.30716e+09\\
1723.47042e-06 1.14137e+09\\
1735.0407e-06 1.13935e+09\\
1747.30873e-06 1.29639e+09\\
1751.05868e-05 1.63104e+09\\
1761.53167e-05 2.18521e+09\\
1772.21346e-05 3.02958e+09\\
1783.19532e-05 4.27201e+09\\
1794.60822e-05 6.07009e+09\\
1806.63988e-05 8.6474e+09\\
1819.55863e-05 1.23123e+10\\
1820.000137462 1.74764e+10\\
1830.00019741 2.46663e+10\\
1840.000282939 3.45144e+10\\
1850.000404337 4.77081e+10\\
1860.000575387 6.48747e+10\\
1870.000813969 8.63927e+10\\
1880.00114233 1.12165e+11\\
1890.00158668 1.41452e+11\\
1900.00217581 1.72884e+11\\
1910.00293882 2.04708e+11\\
1920.00390223 2.35161e+11\\
1930.00508728 2.62745e+11\\
1940.0065082 2.86241e+11\\
1950.00817167 3.04381e+11\\
1960.0100772 3.15206e+11\\
1970.0122182 3.15161e+11\\
1980.0145821 2.98536e+11\\
1990.0171503 2.59523e+11\\
2000.0198959 1.99872e+11\\
2010.0227786 1.35295e+11\\
2020.0257388 8.44329e+10\\
2030.0286968 5.29578e+10\\
2040.0315715 3.59953e+10\\
2050.0343251 2.72259e+10\\
2060.0369758 2.24818e+10\\
2070.0395621 1.96668e+10\\
2080.0421177 1.78143e+10\\
209};
210\addlegendentry{$V_{\mathrm{BC}}=\SI{0.50}{\volt}$}
211\end{axis}
212
213\end{tikzpicture}
214\end{document}