Basic data handling example
The example code
"""
This example shows the basic data handling with python.
We will read data, clean it, calculate with the data and then plot.
"""
from pathlib import Path
from DMT.core import read_data, specifiers, Plot
# path to static folder:
path_static = Path(__file__).resolve().parent.parent / "_static"
# some specifiers, they allow consistent access to electrical data
# all interfaces of DMT adhere to these specifiers!
col_vbe = specifiers.VOLTAGE + ["B", "E"]
col_vbc = specifiers.VOLTAGE + ["B", "C"]
col_ic = specifiers.CURRENT + "C"
col_ib = specifiers.CURRENT + "B"
# read data using the given method
data = read_data(path_static / "HBT_vbc.elpa")
# "clean" the data so we have DMT-specifier in it.
# This ensure that the read-in data is consistent with the DMT specifiers.
# clean data additionally removes all voltages and only potentials remain
data = data.clean_data(["B", "C", "E"], reference_node="E")
# adjust unit from read in file (currents were in mA, DMT uses always basic units):
data[col_ic] = data[col_ic] * 1e-3
data[col_ib] = data[col_ib] * 1e-3
# The "ensure_specifier_column" method can be used to ensure that a given derived
# electrical quantity, such as a voltage, is inside the dataframe. If it is not there,
# an algorithm will try to generate the quantity.
data.ensure_specifier_column(col_vbe)
data.ensure_specifier_column(col_vbc)
# generate the plot object with proper axis and legend location
plot = Plot(
"I_C(V_BE)",
x_specifier=col_vbe,
y_specifier=col_ic,
y_scale=1e3,
y_log=True,
legend_location="lower right",
)
plot.legend_frame = False
plot.x_limits = (0.6, 1.1)
# fill the gummel plot with a line for each V_BC
for _index, vbc, data_vbc in data.iter_unique_col(col_vbc, decimals=3):
plot.add_data_set(
data_vbc[col_vbe],
data_vbc[col_ic],
label="${:s}= \\SI{{{:.1f}}}{{\\volt}}$".format(col_vbc.to_tex(), vbc),
)
# show the plot (DMT has three plotting back-ends: matplotlib, pyqtgraph and tikz)
# plot.plot_pyqtgraph()
plot.plot_py()
# save the plot as tikz and build it
plot.save_tikz(
path_static,
standalone=True,
build=True,
clean=True,
width="3in",
)
The generated files
I_CV_BE.pdf
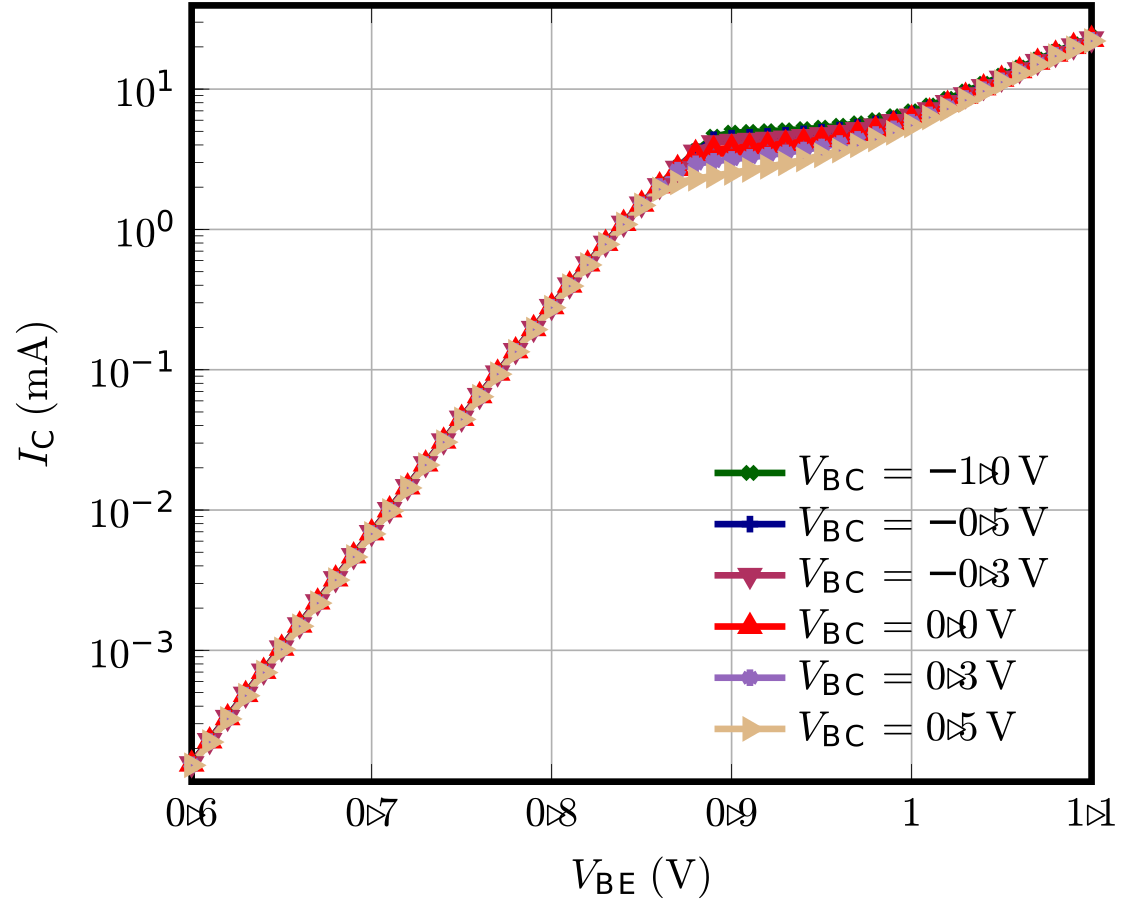
I_CV_BE.tex
1\documentclass[class=IEEEtran]{standalone}
2\usepackage{tikz,amsmath,siunitx}
3\usetikzlibrary{arrows,snakes,backgrounds,patterns,matrix,shapes,fit,calc,shadows,plotmarks}
4\usepackage[graphics,tightpage,active]{preview}
5\usepackage{pgfplots}
6\pgfplotsset{compat=newest}
7\usetikzlibrary{shapes.geometric}
8\PreviewEnvironment{tikzpicture}
9\PreviewEnvironment{equation}
10\PreviewEnvironment{equation*}
11\newlength\figurewidth
12\newlength\figureheight
13\begin{document}
14\setlength\figurewidth{60mm}
15\setlength\figureheight{60mm}
16\begin{tikzpicture}[font=\normalsize]
17\pgfplotsset{every axis/.append style={ultra thick},compat=1.5},
18\definecolor{color0}{rgb}{0.00000, 0.39216, 0.00000}
19\definecolor{color1}{rgb}{0.00000, 0.00000, 0.54510}
20\definecolor{color2}{rgb}{0.69020, 0.18824, 0.37647}
21\definecolor{color3}{rgb}{1.00000, 0.00000, 0.00000}
22\definecolor{color4}{rgb}{0.58039, 0.40392, 0.74118}
23\definecolor{color5}{rgb}{0.87059, 0.72157, 0.52941}
24
25\begin{axis}[scale only axis,ytick pos=left,
26width=3in,
27xlabel={$V_{\mathrm{BE}}\left(\si{\volt}\right)$},
28ylabel={$I_{\mathrm{C}}\left(\si{\milli\ampere}\right)$},
29ymode=log,
30xmin=0.6,
31xmax=1.1,
32restrict x to domain=0.12:5.5,
33log basis x=10,
34ymin=0.000115874,
35ymax=39.5057,
36restrict y to domain=-19.6801:7.9833,
37log basis y=10,
38xmajorgrids,
39enlargelimits=false,
40scaled ticks=true,
41ymajorgrids,
42x tick style={color=black},
43y tick style={color=black},
44x grid style={white!69.01960784313725!black},
45y grid style={white!69.01960784313725!black},
46/tikz/mark repeat=1,
47legend style={at={(0.98,0.02)}, anchor=south east,legend cell align=left, align=left, fill=none, draw=none},
48]
49\addplot [color=color0, solid, mark=x, mark options={solid}, mark phase=1, ]
50 table[row sep=crcr, x expr=\thisrowno{0}*1.000000e+00, y expr=\thisrowno{1}*1.000000e+03]{
510.6 1.57931e-07\\
520.61 2.31036e-07\\
530.62 3.37917e-07\\
540.63 4.94143e-07\\
550.64 7.22433e-07\\
560.65 1.05593e-06\\
570.66 1.54295e-06\\
580.67 2.25389e-06\\
590.68 3.29124e-06\\
600.69 4.80404e-06\\
610.7 7.00881e-06\\
620.71 1.02196e-05\\
630.72 1.4891e-05\\
640.73 2.16796e-05\\
650.74 3.15304e-05\\
660.75 4.57977e-05\\
670.76 6.64121e-05\\
680.77 9.61046e-05\\
690.78 0.000138701\\
700.79 0.00019949\\
710.8 0.000285662\\
720.81 0.000406776\\
730.82 0.000575186\\
740.83 0.000806309\\
750.84 0.00111858\\
760.85 0.00153297\\
770.86 0.00207183\\
780.87 0.002757\\
790.88 0.00360467\\
800.89 0.0045765\\
810.9 0.00488923\\
820.91 0.00494777\\
830.92 0.0050081\\
840.93 0.00508284\\
850.94 0.00517917\\
860.95 0.00530589\\
870.96 0.00547472\\
880.97 0.00569853\\
890.98 0.00599919\\
900.99 0.00640369\\
911 0.00694022\\
921.01 0.00763601\\
931.02 0.00851569\\
941.03 0.00959848\\
951.04 0.0109032\\
961.05 0.0124441\\
971.06 0.0142227\\
981.07 0.016231\\
991.08 0.0184492\\
1001.09 0.0208371\\
1011.1 0.023341\\
102};
103\addlegendentry{$V_{\mathrm{BC}}= \SI{-1.0}{\volt}$}
104\addplot [color=color1, solid, mark=+, mark options={solid}, mark phase=1, ]
105 table[row sep=crcr, x expr=\thisrowno{0}*1.000000e+00, y expr=\thisrowno{1}*1.000000e+03]{
1060.6 1.56378e-07\\
1070.61 2.28783e-07\\
1080.62 3.34651e-07\\
1090.63 4.8941e-07\\
1100.64 7.15578e-07\\
1110.65 1.04601e-06\\
1120.66 1.5286e-06\\
1130.67 2.23314e-06\\
1140.68 3.26127e-06\\
1150.69 4.76079e-06\\
1160.7 6.94645e-06\\
1170.71 1.01298e-05\\
1180.72 1.47619e-05\\
1190.73 2.14943e-05\\
1200.74 3.1265e-05\\
1210.75 4.54186e-05\\
1220.76 6.58721e-05\\
1230.77 9.53386e-05\\
1240.78 0.000137619\\
1250.79 0.000197973\\
1260.8 0.000283549\\
1270.81 0.000403858\\
1280.82 0.0005712\\
1290.83 0.000800931\\
1300.84 0.00111142\\
1310.85 0.00152352\\
1320.86 0.00205942\\
1330.87 0.00274027\\
1340.88 0.00357795\\
1350.89 0.00435599\\
1360.9 0.00447169\\
1370.91 0.00453578\\
1380.92 0.00460511\\
1390.93 0.00468889\\
1400.94 0.00479386\\
1410.95 0.00492834\\
1420.96 0.00510194\\
1430.97 0.00532997\\
1440.98 0.00563537\\
1450.99 0.00604573\\
1461 0.00659001\\
1471.01 0.00729441\\
1481.02 0.00818138\\
1491.03 0.00927128\\
1501.04 0.0105796\\
1511.05 0.0121198\\
1521.06 0.0138983\\
1531.07 0.0159047\\
1541.08 0.0181156\\
1551.09 0.0204957\\
1561.1 0.0229951\\
157};
158\addlegendentry{$V_{\mathrm{BC}}= \SI{-0.5}{\volt}$}
159\addplot [color=color2, solid, mark=triangle, mark options={solid, rotate=180}, mark phase=1, ]
160 table[row sep=crcr, x expr=\thisrowno{0}*1.000000e+00, y expr=\thisrowno{1}*1.000000e+03]{
1610.6 1.55669e-07\\
1620.61 2.27755e-07\\
1630.62 3.3316e-07\\
1640.63 4.8725e-07\\
1650.64 7.12449e-07\\
1660.65 1.04148e-06\\
1670.66 1.52204e-06\\
1680.67 2.22367e-06\\
1690.68 3.24757e-06\\
1700.69 4.74102e-06\\
1710.7 6.91795e-06\\
1720.71 1.00888e-05\\
1730.72 1.47029e-05\\
1740.73 2.14096e-05\\
1750.74 3.11435e-05\\
1760.75 4.52449e-05\\
1770.76 6.56247e-05\\
1780.77 9.49873e-05\\
1790.78 0.000137123\\
1800.79 0.000197275\\
1810.8 0.000282575\\
1820.81 0.00040251\\
1830.82 0.000569351\\
1840.83 0.000798421\\
1850.84 0.00110804\\
1860.85 0.001519\\
1870.86 0.00205332\\
1880.87 0.00273153\\
1890.88 0.00355995\\
1900.89 0.00415437\\
1910.9 0.0042477\\
1920.91 0.00431721\\
1930.92 0.00439326\\
1940.93 0.00448412\\
1950.94 0.00459637\\
1960.95 0.00473761\\
1970.96 0.00491714\\
1980.97 0.00515087\\
1990.98 0.00546182\\
2000.99 0.00587797\\
2011 0.00642824\\
2021.01 0.00713864\\
2031.02 0.0080306\\
2041.03 0.00912394\\
2051.04 0.010435\\
2061.05 0.0119757\\
2071.06 0.0137532\\
2081.07 0.0157592\\
2091.08 0.0179685\\
2101.09 0.0203448\\
2111.1 0.0228409\\
212};
213\addlegendentry{$V_{\mathrm{BC}}= \SI{-0.3}{\volt}$}
214\addplot [color=color3, solid, mark=triangle, mark options={solid, rotate=0}, mark phase=1, ]
215 table[row sep=crcr, x expr=\thisrowno{0}*1.000000e+00, y expr=\thisrowno{1}*1.000000e+03]{
2160.6 1.54461e-07\\
2170.61 2.26002e-07\\
2180.62 3.30619e-07\\
2190.63 4.83566e-07\\
2200.64 7.07112e-07\\
2210.65 1.03375e-06\\
2220.66 1.51086e-06\\
2230.67 2.20749e-06\\
2240.68 3.2242e-06\\
2250.69 4.70728e-06\\
2260.7 6.86928e-06\\
2270.71 1.00186e-05\\
2280.72 1.4602e-05\\
2290.73 2.12647e-05\\
2300.74 3.09358e-05\\
2310.75 4.49479e-05\\
2320.76 6.52011e-05\\
2330.77 9.43853e-05\\
2340.78 0.000136271\\
2350.79 0.000196076\\
2360.8 0.000280897\\
2370.81 0.00040018\\
2380.82 0.00056614\\
2390.83 0.000794028\\
2400.84 0.00110206\\
2410.85 0.00151084\\
2420.86 0.00204185\\
2430.87 0.00271311\\
2440.88 0.0034726\\
2450.89 0.00371751\\
2460.9 0.00380651\\
2470.91 0.00388909\\
2480.92 0.00398065\\
2490.93 0.00408838\\
2500.94 0.00421844\\
2510.95 0.00437727\\
2520.96 0.0045744\\
2530.97 0.00482538\\
2540.98 0.00515277\\
2550.99 0.0055848\\
2561 0.00615026\\
2571.01 0.00687402\\
2581.02 0.00777703\\
2591.03 0.00887756\\
2601.04 0.0101932\\
2611.05 0.0117365\\
2621.06 0.0135125\\
2631.07 0.0155162\\
2641.08 0.0177239\\
2651.09 0.0200962\\
2661.1 0.0225853\\
267};
268\addlegendentry{$V_{\mathrm{BC}}= \SI{0.0}{\volt}$}
269\addplot [color=color4, solid, mark=asterisk, mark options={solid}, mark phase=1, ]
270 table[row sep=crcr, x expr=\thisrowno{0}*1.000000e+00, y expr=\thisrowno{1}*1.000000e+03]{
2710.6 1.52964e-07\\
2720.61 2.2383e-07\\
2730.62 3.27468e-07\\
2740.63 4.78998e-07\\
2750.64 7.00492e-07\\
2760.65 1.02416e-06\\
2770.66 1.49698e-06\\
2780.67 2.18742e-06\\
2790.68 3.19518e-06\\
2800.69 4.66537e-06\\
2810.7 6.80881e-06\\
2820.71 9.93149e-06\\
2830.72 1.44766e-05\\
2840.73 2.10844e-05\\
2850.74 3.06771e-05\\
2860.75 4.45776e-05\\
2870.76 6.46723e-05\\
2880.77 9.36325e-05\\
2890.78 0.000135203\\
2900.79 0.000194567\\
2910.8 0.000278776\\
2920.81 0.000397211\\
2930.82 0.000562003\\
2940.83 0.000788269\\
2950.84 0.00109399\\
2960.85 0.00149917\\
2970.86 0.00202287\\
2980.87 0.00264508\\
2990.88 0.00293828\\
3000.89 0.00305683\\
3010.9 0.00315725\\
3020.91 0.00326133\\
3030.92 0.00337801\\
3040.93 0.00351352\\
3050.94 0.00367362\\
3060.95 0.00386512\\
3070.96 0.00409744\\
3080.97 0.00438453\\
3090.98 0.00474703\\
3100.99 0.0052115\\
3111 0.00580521\\
3121.01 0.00655175\\
3131.02 0.00747146\\
3141.03 0.00858347\\
3151.04 0.00990534\\
3161.05 0.0114516\\
3171.06 0.0132283\\
3181.07 0.0152289\\
3191.08 0.0174323\\
3201.09 0.0198004\\
3211.1 0.0222831\\
322};
323\addlegendentry{$V_{\mathrm{BC}}= \SI{0.3}{\volt}$}
324\addplot [color=color5, solid, mark=triangle, mark options={solid, rotate=270}, mark phase=1, ]
325 table[row sep=crcr, x expr=\thisrowno{0}*1.000000e+00, y expr=\thisrowno{1}*1.000000e+03]{
3260.6 1.51634e-07\\
3270.61 2.21907e-07\\
3280.62 3.24685e-07\\
3290.63 4.74968e-07\\
3300.64 6.94657e-07\\
3310.65 1.01571e-06\\
3320.66 1.48476e-06\\
3330.67 2.16974e-06\\
3340.68 3.16963e-06\\
3350.69 4.62845e-06\\
3360.7 6.75553e-06\\
3370.71 9.85468e-06\\
3380.72 1.4366e-05\\
3390.73 2.09253e-05\\
3400.74 3.04486e-05\\
3410.75 4.425e-05\\
3420.76 6.42035e-05\\
3430.77 9.2963e-05\\
3440.78 0.000134249\\
3450.79 0.000193211\\
3460.8 0.00027685\\
3470.81 0.000394476\\
3480.82 0.0005581\\
3490.83 0.000782617\\
3500.84 0.00108542\\
3510.85 0.00148359\\
3520.86 0.00193031\\
3530.87 0.00216209\\
3540.88 0.00229712\\
3550.89 0.00241257\\
3560.9 0.00252828\\
3570.91 0.0026535\\
3580.92 0.00279469\\
3590.93 0.00295766\\
3600.94 0.00314863\\
3610.95 0.00337497\\
3620.96 0.00364612\\
3630.97 0.003975\\
3640.98 0.00437957\\
3650.99 0.00488256\\
3661 0.00550803\\
3671.01 0.00627846\\
3681.02 0.00721484\\
3691.03 0.00833756\\
3701.04 0.00966576\\
3711.05 0.0112146\\
3721.06 0.0129913\\
3731.07 0.0149899\\
3741.08 0.0171885\\
3751.09 0.01955\\
3761.1 0.0220256\\
377};
378\addlegendentry{$V_{\mathrm{BC}}= \SI{0.5}{\volt}$}
379\end{axis}
380
381\end{tikzpicture}
382\end{document}